Kotlin has become the preferred language for Android app development, thanks to its simplicity, safety, and powerful features. If you're a beginner, this guide will walk you through setting up your development environment, creating your first Kotlin project, and introducing you to the basics of the Kotlin language. You'll learn how to:
By the end of this guide, you'll have a solid foundation for developing Android apps using Kotlin, and be ready to explore more advanced features and concepts.
Downloading and Installing Android Studio
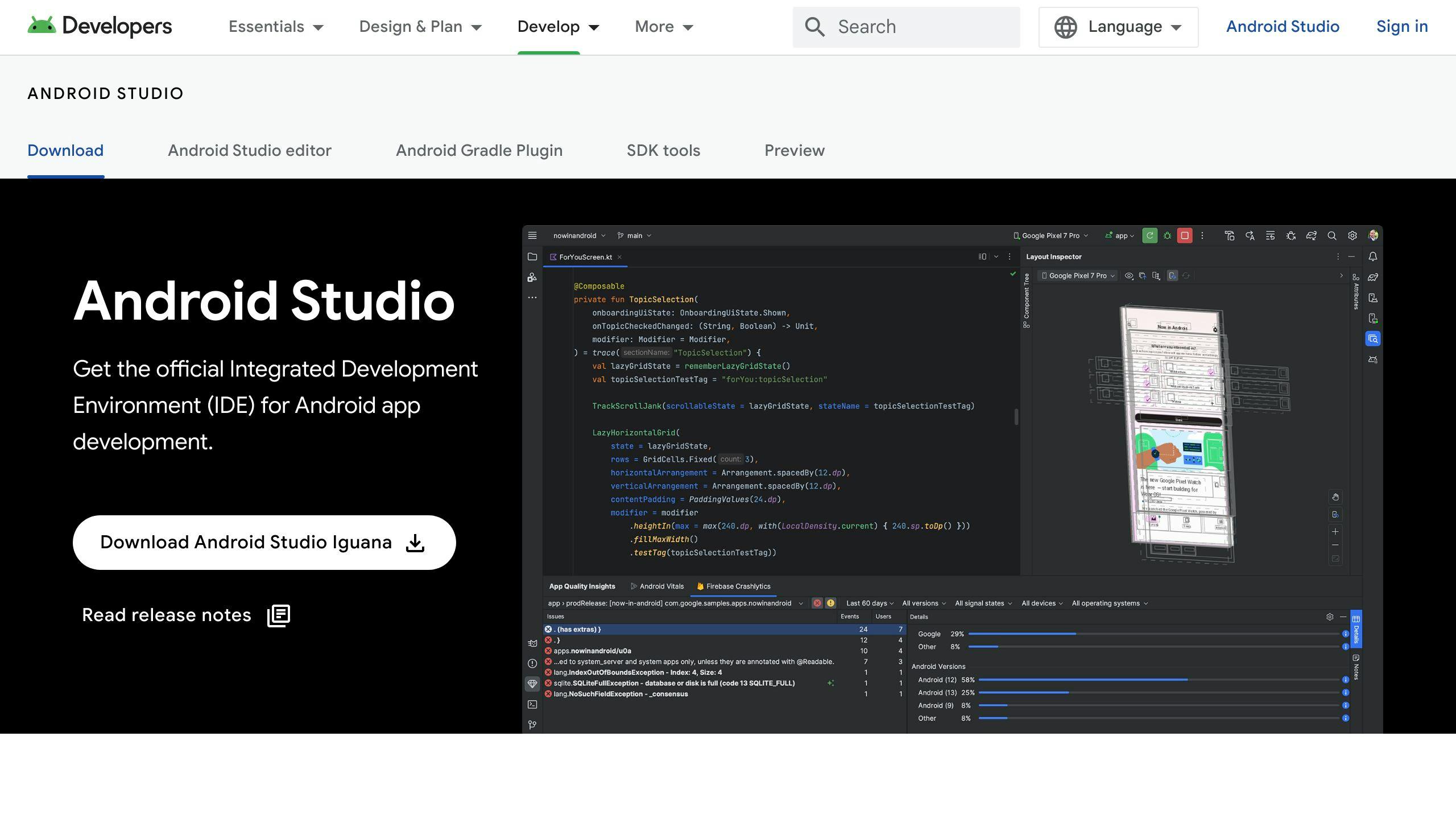
To kick off making Android apps with Kotlin, the first step is getting Android Studio on your computer. It's the main tool that Google offers for Android app development. Here's how to do it:
When it's all done, Android Studio will open up, and you're ready to start building your first app with Kotlin.
Creating an Android Virtual Device (AVD)
An Android Virtual Device (AVD) lets you test your apps without needing a real Android phone. Setting one up is pretty straight forward:
Now, you've got a virtual phone in the AVD Manager ready to test your apps.
Creating Your First Kotlin Project

Creating a New Project
To start making your first app with Kotlin in Android Studio, follow these steps:
This step creates a new project where Kotlin is the main language, including some basic setup.
Touring the Default Project Files
Your new project will have several important files:
Take a moment to look through these to get a feel for the project layout.
Modifying the Default TextView
To change the initial screen text to "Hello World":
Running the App
To see your app in action, connect an emulator or a real device via USB and press the run button. You should see the "Hello World" text on the app's screen.
And that's it! You've just made a simple app with Kotlin in Android Studio.
Kotlin Language Basics

Let's dive into the basics of Kotlin, the language you'll use to create Android apps. We'll keep it simple and clear, so you can get a good start.
Key Features of Kotlin
Kotlin has some cool features that make it awesome for making Android apps:
These features help make your Android apps strong, simple, and fast.
Variables and Data Types
In Kotlin, you use var
for things that can change and val
for things that don't:
var mutableInt = 5 // This can change
val readOnlyString = "Hello" // This can't change
Kotlin uses common types like:
Control Flow Statements
Kotlin has easy ways to make decisions in your code:
Conditional - if/else
if(age < 18) {
print("Underage")
}
else {
print("Adult")
}
When expression - This is like choosing based on a condition. It's similar to switch/case in other languages.
when(color) {
"Red" -> print("Stop")
"Green" -> print("Go")
else -> print("Slow down")
}
Loops - for/while
for(item in list) {
print(item)
}
while(x > 5) {
x--
}
These help you run code based on conditions or repeat actions.
Functions in Kotlin
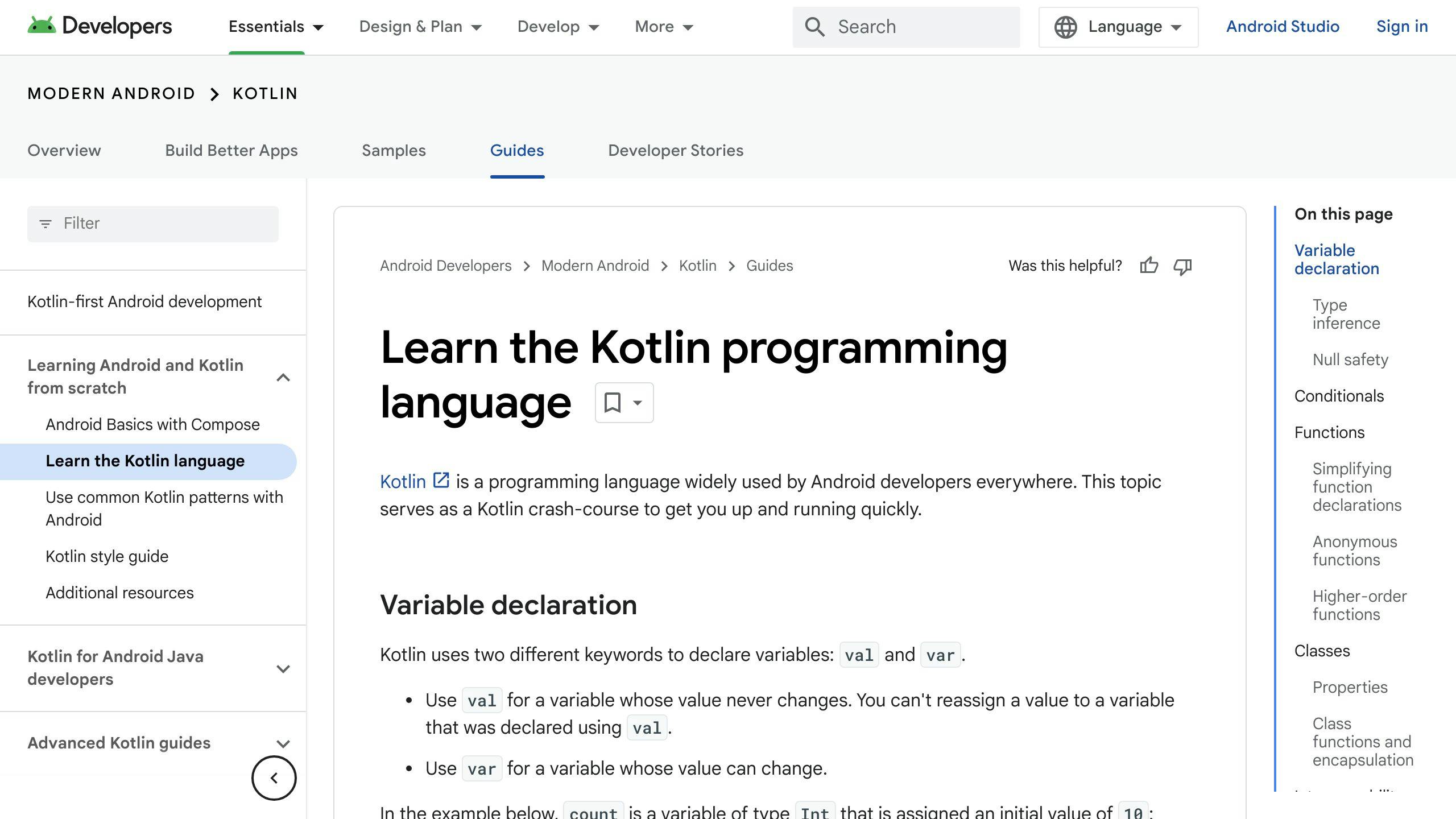
To do something in Kotlin, you write functions. Here's how:
fun add(a: Int, b: Int): Int {
return a + b
}
Getting these basics down is a great start to making your own Android apps with Kotlin.
Building a Simple User Interface
In this part, we'll show you how to make a simple screen with a button that you can press and a spot where text shows up. We'll use Android's design tools and some Kotlin code to do this.
Adding UI Elements in Layout XML

First, we'll layout our screen in an XML file. We'll use a LinearLayout
that stacks our elements vertically, including a TextView
for showing messages, and a Button
for user clicks:
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me"/>
</LinearLayout>
Here, we're setting up how big each element is, what it says, and giving each one a unique ID.
Wiring Up Button Click Handler
Next, in our main activity Kotlin file, we find our button and tell it what to do when clicked:
val button = findViewById<Button>(R.id.button)
button.setOnClickListener {
// Handle click
}
This code makes sure something happens when the button is pressed.
Updating Text when Button Clicked
Inside the block of code that handles clicks, we can change what our text view says:
val textView = findViewById<TextView>(R.id.text_view)
button.setOnClickListener {
textView.text = "Button clicked!"
}
Now, whenever the button is clicked, the text on the screen will change to let the user know. It's a simple way to interact with your app.
sbb-itb-8abf120
Running and Testing Your App
Trying Out Your App
After you've built your app with Kotlin, it's time to see it in action. Here's how you can test it:
To run your Kotlin app:
Testing your app well, both by trying it yourself and using tests, is important before you share it with others.
Using Logcat for Debugging
Android Studio's Logcat tool lets you see messages from your app while it's running. This is super helpful for figuring out problems.
To add a message in your code, use:
Log.d("MainActivity", "Button clicked")
Then, when you run your app, you can see this message in Logcat. It's great for understanding what's happening in your app, especially when something goes wrong.
Running UI Tests
Espresso is a tool for testing how your app looks and works. You can write tests that check things like whether tapping a button changes the text on the screen.
Here's an example test:
@Test
fun button_click_updatesText() {
// Pretend to tap the button
onView(withId(R.id.button)).perform(click())
// Check if the text changed correctly
onView(withId(R.id.text_view)).check(matches(withText("Button clicked!")))
}
This test makes sure that when you tap the button, the text changes as expected. Adding tests like this for the main things your app does is a good way to make sure everything works right, even when you make changes later on.
Next Steps
After you've started with Kotlin and made your first Android app, there's much more you can learn and do. Here are some next steps to take in your journey of making Android apps:
Storing Data
Apps often need to keep track of information like what settings a user prefers or what content they've downloaded. Kotlin makes it easy to work with different ways of saving data:
Connecting to the Internet
Many apps get information from the internet. Kotlin works well with some popular tools for this:
Advanced Functionality
Kotlin lets you add cool features to your apps:
Architecture Patterns
When your app gets bigger, it's important to keep your code organized. Using patterns like:
Can help you manage more complex apps.
Kotlin and Android are always getting better. There's always something new to learn! By keeping up with Android's updates and reading about new features, you can make your apps better and do more cool things with them.
Related Questions
Is Kotlin good for mobile app development?
Yes, Kotlin is a top choice for making apps, especially for Android. It's made to fix common problems and make coding easier. Here's why Kotlin is great for app making:
Most Android developers prefer Kotlin because it makes their work better and easier.
Is Kotlin alone enough for Android development?
Yes, Kotlin has everything you need to make Android apps from start to finish. It includes:
Kotlin's rich set of tools and libraries means you can build complete, high-quality apps just with Kotlin.
How much time will it take to learn Kotlin for Android app development?
If you already know how to program, you can pick up the basics of Kotlin in 2-4 weeks. You might even start making simple apps in the first week. But to really get good at Kotlin and Android, you'll need 3-6 months if you practice by making apps.
The best way to learn fast is by actually building apps, not just reading about how to do it. This hands-on practice helps you learn quicker.
How do I create a mobile app using Kotlin?
Here's a quick guide to making your first Android app with Kotlin:
Look for courses or books on Kotlin Android app development for detailed steps. The more you practice making apps, the better you'll get.