If you're curious about Flutter, Google's UI toolkit for crafting natively compiled applications for mobile, web, and desktop from a single codebase, you've come to the right place. This comprehensive guide covers everything from Flutter's origins and core capabilities to its architecture, UI components, state management, testing, and how to deploy apps. Here's what you need to know:
Whether you're a beginner or an experienced developer, this guide offers valuable insights into making the most of Flutter for your projects.
Origin and History
Google first talked about Flutter in May 2017 at a big event for developers. They released a test version that year, and by 2018, Flutter 1.0 was ready for everyone. Since then, it has gotten better and more popular, with the latest version being Flutter 2.8.
Overview
Think of Flutter as a big box of tools and parts for making apps that look good and work well on different devices. It's special because:
Core Capabilities
Here's what makes Flutter really good for making apps:
Adoption and Community
Flutter is becoming more popular:
Flutter also has a strong community with lots of support, which helps more people learn how to use it.
Why Choose Flutter?
Flutter stands out because it makes creating mobile apps easier, quicker, and more efficient. It's great for making apps that work on both iPhones and Android phones using the same set of instructions. Let's dive into some of the reasons why Flutter is a smart choice.
Single Codebase
The coolest thing about Flutter is that you only need to write your app's code once, and then it can run on different devices. This means you don't have to write separate codes for iOS and Android, saving you a lot of time and effort.
This approach is especially helpful for smaller teams or startups. They can make apps for both major platforms without needing more people or spending more money. Flutter makes it possible to do more with less.
Comparison Table
MetricNative (Java/Kotlin, Swift/Obj-C)FlutterDevelopment TimeHigh (develop twice)Fast (single codebase)Code SharingNone100% sharedPerformanceNative (direct)Near-native (compiled)UI FlexibilityPlatform-dependentHighly customizableTestingPlatform-specificUnified framework
This table shows that Flutter lets you build apps faster, share code, and create custom looks while keeping the app running smoothly.
Customizable UI
Flutter gives you lots of tools to make your app look just the way you want. You can change colors, styles, and layouts to match your brand, and it's easy to make your app look good on all kinds of devices, from phones to tablets to computers.
This means you can get creative with your app's design, making sure it stands out and fits your brand perfectly.
Testing Capabilities
Flutter also has great tools for checking that your app works well. You can test small parts, how the app looks, and even the whole app from start to finish. This helps you catch any problems early and make sure your app is as good as it can be before anyone else sees it.
Testing makes it easier to try new things and update your app without worrying about breaking it. This way, you can keep making your app better and better over time.
Overall, Flutter is a great choice for making mobile apps because it saves time, lets you get creative with your design, and helps you make sure your app works well.
Architecture of Flutter
Flutter is built with a design that separates its parts into layers, making it easier to handle different tasks. Let's break down these layers:
Framework
The core of Flutter that you interact with is made using a programming language called Dart. Here are some key parts of this layer:
Engine
This part deals with the heavy lifting and is written in C++. Here's what it does:
Custom Flutter Engine Embedders
To make a Flutter app work on a device like a phone or computer, the engine uses something called embedders. These help with:
In short, Flutter's design is smart because it separates tasks into different layers. This setup helps developers make apps more easily, allows for customization, and keeps apps running fast. The way Flutter connects with devices also makes sure your app can do what it needs to on whatever device it's on.
Building Blocks of Flutter UI
Widgets
Widgets are like the Lego pieces of a Flutter app's screen. They help you show what you want on the app, like buttons, text, or images. If something changes in the app, like a new message popping up, widgets update themselves to show the new info.
Here are some things to know about widgets:
Widgets connect to each other like a family tree, and changing one can affect others linked to it.
Layout
Flutter lets you arrange your widgets in many ways, like in a line up and down (Column), side to side (Row), stacked on top of each other (Stack), or inside a box (Container) that can have space around it or a border.
There are also special widgets for making lists, grids, and things that scroll.
Navigation and Routing
Flutter uses a map-like system for moving between screens in the app. It uses a Navigator widget that works like a stack of cards, where you can add a new screen on top or remove the top one to go back.
You can also pass info from one screen to another, which is handy for things like opening a detailed view from a list.
Gesture Support
Flutter makes it easy to deal with taps, swipes, and pinches in your app with widgets designed for catching these gestures. This makes your app feel smooth and natural to use.
You can even make your own gestures if you need something special.
In short, Flutter gives you all the tools to make your app look good and work well, from putting things on the screen to making them interactive. It's great for making mobile apps quickly and efficiently.
State Management in Flutter
Managing how your app keeps track of changes and updates its look is key in making a good Flutter app. Here are some ways to handle state management in Flutter:
Local State Management
Flutter lets you manage simple state changes right inside your widgets using StatefulWidgets and a method called setState()
.
BLoC Pattern
The BLoC (Business Logic Component) pattern helps keep your app's logic separate from its look.
Redux

Redux is a system that helps manage your app's state in a predictable way, following a one-way data flow.
MobX
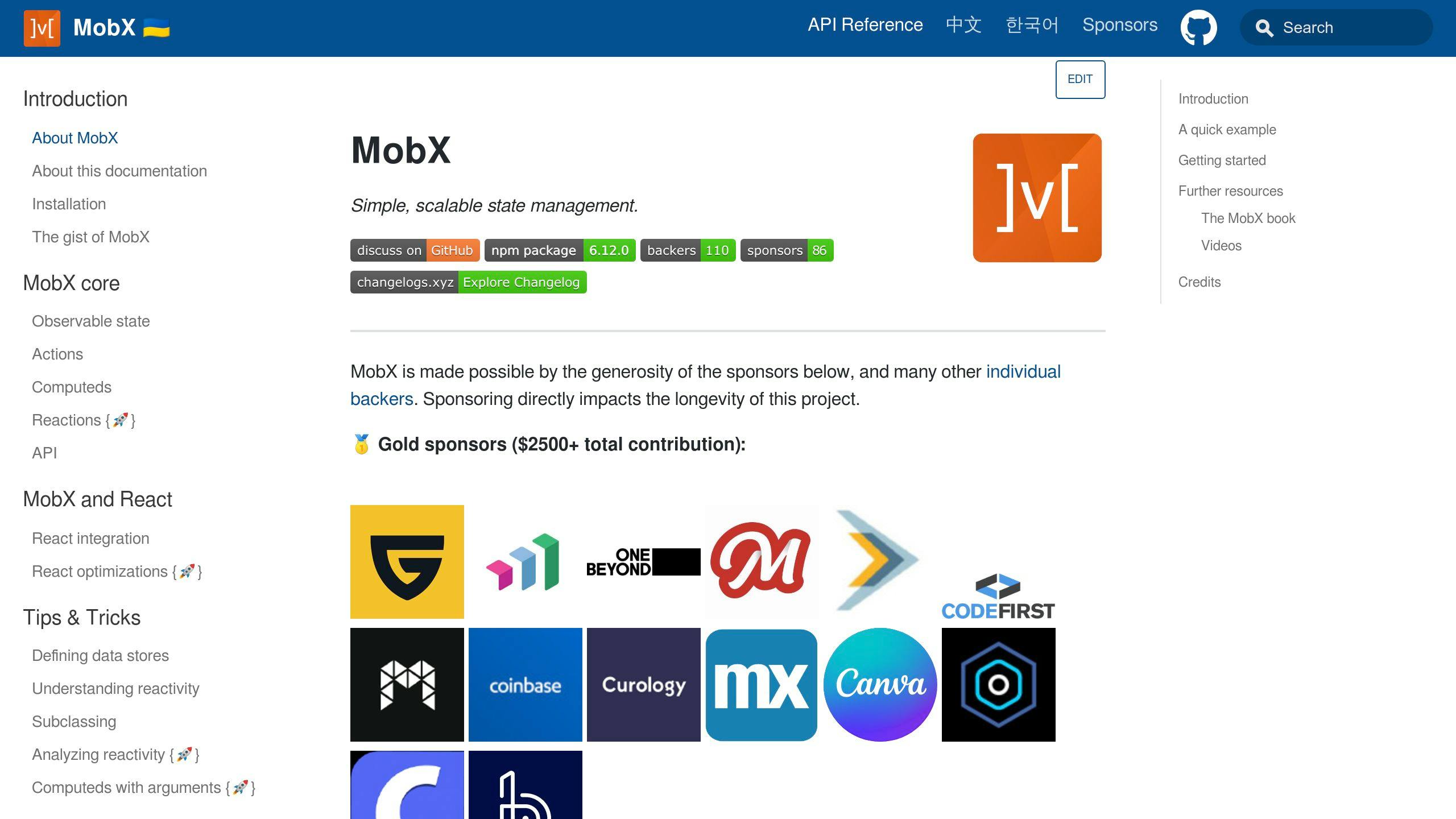
MobX uses a system of observables and reactions to handle state changes.
In the end, each method has its pros and cons. Think about what your app needs to decide which state management approach is the best fit.
sbb-itb-8abf120
Testing Flutter Apps
Testing is super important when you're making apps with Flutter. It helps make sure your app works well and doesn't have any big problems. Let's look at the different ways you can test your Flutter apps.
Unit Testing
Unit testing is when you check small parts of your app, like a single function or widget, to see if they do what they're supposed to do.
With Flutter, you can use something called flutter_test
to do unit tests. This means you can check parts of your app by themselves to make sure they're working right.
Things you might want to test include:
Widget Testing
Widget testing is all about making sure the visual parts of your app look and work the way they should, like making sure a button shows up correctly and can be tapped.
You should use widget tests to check that each piece of your app's design is good to go on its own before you add it to the whole app. Flutter's flutter_test
package helps you do this.
Integration Testing
Integration testing is when you test big parts of your app to see if different sections work well together.
Use integration tests to make sure the main things your app is supposed to do work all the way through. Flutter has tools for these kinds of tests, too, so you can run your app and check it automatically.
Doing all these tests helps you find and fix problems early, keep your app running well, and make sure it's as good as it can be before people start using it.
Native Capabilities via Platform Channels
Flutter lets you use features that are specific to iPhones and Android phones through something called platform channels. This is handy for using things that are built into the phone, like the camera or sensor features, or for showing parts of the app that look just right on each type of phone.
Method Channels
Method channels are a way for the Flutter app to talk to the phone's own system and ask it to do something.
Here's a simple way to use the camera:
static const platform = MethodChannel('com.example.app/camera');
String path = await platform.invokeMethod('takePicture');
Method channels are straightforward for tasks that just need to happen once.
Event Channels
Event channels let your app know about ongoing activities from the phone, like moving around or updates from sensors.
This is how you can keep up with where the user is:
EventChannel locationStream = EventChannel('com.example.app/location');
locationStream.receiveBroadcastStream().listen((event) {
// Change the app based on new location
});
Event channels are your go-to for continuous updates from the phone.
Embedding Native Views
Flutter can also show parts of the app using the phone's own design elements.
Here's how to show a map the Android way:
AndroidView(
viewType: 'maps',
onPlatformViewCreated: (viewId) {
// Work with the native map
},
);
Using embedded views is a smart choice when you need the app to have parts that look exactly like they do on the phone.
Building and Deploying Flutter Apps
Android Build Process
To make your Flutter app ready for Android users, you can use these simple steps:
This process does a few important things:
Flutter's tools help you get your app ready for Android without much hassle.
iOS Build Process
For getting your Flutter app on iOS devices:
Here's what happens during this:
You need to use Xcode to make sure your iOS app meets all the requirements for the App Store.
Releasing to App Stores
When you're ready to share your Flutter app with the world:
Both the Play Store and App Store have rules about what your app can do and what information you need to provide. Make sure to look at their guidelines to get your app approved.
Conclusion
Flutter is a great tool for making both mobile and web apps quickly and without too much hassle. It comes packed with features that make it easy for anyone to create good-looking and fast apps.
Here's a quick rundown of what makes Flutter special:
Cross-platform development
Rapid iteration
Expressive and flexible UI
Near-native performance
Testing capabilities
Community and ecosystem
Flutter makes it simpler to build apps that look and work great on different devices. As more people start using it, it's likely to become an even more popular choice for creating apps quickly and efficiently.
Related Questions
What is the best framework for Flutter?
When it comes to making Flutter mobile apps, some frameworks stand out because they help manage how your app works and looks. Here are a few:
Choosing the right one depends on what your app needs. GetX and BLoC are good places to start for most apps. Redux is better for big, complex apps, and MobX makes managing changes easy.
Is Flutter framework good?
Yes, Flutter is really good for building apps. Here's why:
Flutter is popular because it's fast and flexible, making it a favorite for app development.
How much does Flutter framework cost?
The cost to make a Flutter app can change a lot based on what you want your app to do. Here are some rough ideas:
The price changes based on how much work is needed. Simple apps with just a few features are cheaper than ones with lots of complicated parts. Remember, after your app is made, you'll also need to spend money to keep it running smoothly.
Is Flutter a GUI framework?
Yes, Flutter is a framework designed to help you make the visual part of your app. It's full of tools and widgets that make designing and building your app's interface easier.
Flutter is great for creating apps that look and work well on both mobile phones and the web. It does a lot of the heavy lifting for you, making it simpler to make apps that feel natural on any device.